In the Google Group Apps Script community there was an interesting question about returning a Google Calendar event owner/organiser. The problem was that using the Calendar Service it is possible to use getGuestList(includeOwner)
to return the EventGuest[]
array and in theory using getGuestStatus()
find the guest with the OWNER
GuestStatus
Enum
:
const event = CalendarApp.getEventById('some_event_id'); const guestList = event.getGuestList(true); // get the guest list including owner // Iterate across EventGuest[] for (let i = 0; i < guestList.length; i++){ let guest = guestList[i].getEmail(); let status = guestList[i].getGuestStatus().toString(); Logger.log(guest + ' ' + status); }
However, in practice as the organiser status defaults to ‘Yes’ and can change to ‘No’ or ‘Maybe’ the OWNER status is never returned:

How to solve? Well one solution to find the owner is to get the event guest list with and without the owner then filter out the list ignoring any accounts that appear twice:
const event = CalendarApp.getEventById('some_event_id); const guestList = event.getGuestList(true); // get the guest list including owner const guestListWithoutOwner = event.getGuestList(); // get the guest list without the owner // filter the guest list to ingore duplicate emails leaving only the owner // based on https://stackoverflow.com/a/42995029/1027723 const owner = guestList.filter(o1 => !guestListWithoutOwner.some(o2 => o1.getEmail() === o2.getEmail())); Logger.log('The owner is: ' + owner[0].getEmail());
Alternatively if the Calendar Advanced Service is enabled in your Apps Script project the owner email can be returned using:
// With Calendar Advanced Service enabled // Note: eventId is different to the one returned by CalendarApp as it doesn't include @google.com // See https://stackoverflow.com/a/55545091/1027723 for how to handle const event = Calendar.Events.get(CalendarApp.getDefaultCalendar().getId(), 'some_event_id'); Logger.log('The owner is: ' + event.organizer.email);
Clearly the second method is more direct, but which is quicker? I’ll let you tell me :)
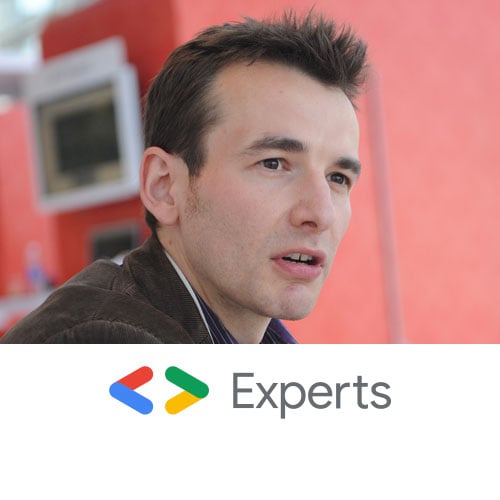
Member of Google Developers Experts Program for Google Workspace (Google Apps Script) and interested in supporting Google Workspace Devs.