If you have tried to seamlessly subscribe a user to a Google Calendar as part of an automation workflow in Google Apps Script and discovered that all that happens is that the user gets an automated email request to join, and then it is up to them to accept the calendar invitation to add it to their live calendar list, you’re in the right place. … How to force subscribe a user in your domain to a Google Calendar using Google Apps Script and Service Accounts.
Scott Donald always crams in lots of very useful tips and guidance in his posts. In this latest piece by Scott you as well as learning about the Google Calendar Advanced Service you can also learn about service accounts and domain wide delegation setup, which enables super powers (and responsibilities). If you are interested in learning more about service accounts in Google Cloud Console Scott has included a selection of other community contributions at the end of his post.
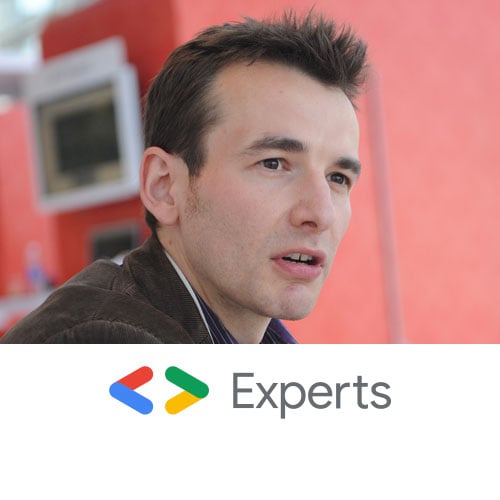
Member of Google Developers Experts Program for Google Workspace (Google Apps Script) and interested in supporting Google Workspace Devs.