As powerful as Google’s new AI tools are, those of us who live in Google Workspace have likely encountered some of their current limitations. One of the most significant for me is in Google Chat. While Gemini can analyse recent conversations, it seems to be limited to roughly the last 20 messages or 7 days of history. Crucially, it also can’t see or process messages sent by apps or webhooks, which are often the lifeblood of a technical team’s space.
This creates a knowledge gap. How can you analyse project history, debug an automated alert, or get a complete overview of a conversation if your tools only see a fraction of the data?
To solve this I’ve created an automated solution using Google Apps Script. This tool can archive an entire Google Chat space history into two powerful, synchronised formats:
- A structured JSON file that serves as the definitive, machine-readable “source of truth”.
- A clean, human-readable Google Doc, which is automatically generated from the JSON data.
This dual-output approach provides the best of both worlds: a portable data file perfect for backups or analysis, and a browsable document ideal for quick searches or for use as a direct data source in a Gemini Gem or other tools like NotebookLM.
Best of all, once configured, you can set up a time-driven trigger to run the script automatically, ensuring your archives are always kept up-to-date without any manual intervention.
Prerequisites
Before you begin, please ensure you have the following:
- A Google Workspace Account: For setup this can only be a Google Workspace account, once it is configured it is possible to test with a personal consumer Gmail account.
- Access to Google Cloud Platform (GCP): You will need the ability to create a new GCP project and enable APIs. The guide below will walk you through creating a fresh project, but if you are using a Google Workspace account, you may need to request a project depending on your organisation’s policies.
- User-Based Access: This script runs as you, the user who authorises it. Therefore, it will only be able to see and archive Google Chat spaces that you are a member of.
The Setup Guide
Getting started is straightforward. The core logic is pre-packaged in a Google Sheet template. You just need to make a copy and connect it to your own Google Cloud project.
Step 1: Copy the Google Sheet Template
First, make your own personal copy of the Chat Archiver template.
➡️ Make a Copy of the Chat Archiver Template ⬅️
Step 2: Configure Your Google Cloud Project (GCP)
This is the most involved step, but it’s essential for giving your new sheet the permission it needs to access your Chat spaces.
- Create a Standard GCP Project:
- Go to the Google Cloud Console.
- Create a new project. Give it a memorable name (e.g., “My Chat Archiver”).
- Enable the Google Chat API:
- In your new project, navigate to the “APIs & Services” > “Enabled APIs & services” and enable the Google Chat API and Google Drive API , or Enable the APIs
- Configure the Chat App (Crucial Step):
- After enabling the API, you’ll be on the Google Chat API page. Click on the Configuration tab. Go to Chat API Configuration page
- Fill in the required details to give your script an identity:
- App Name: e.g., Chat Archiver
- Avatar URL: A public URL to an icon. You can use a placeholder like https://www.gstatic.com/images/icons/material/system/2x/archive\_black\_48dp.png
- Description: e.g., A tool to archive Google Chat spaces.
- Under Interactive features, click the Enable interactive features toggle to the off position to disable interactive features for the Chat app.
- Click Save.
- Configure the OAuth Consent Screen:
- Google Auth platform > Branding. Go to Branding
- If you see a message that says Google Auth platform not configured yet, click Get Started
- Add an App name and support email
- Choose a user type. Internal is best if you’re on a Workspace account. External will work for anyone but require you to add yourself as a test user (you can add up to 100 testers without the need of going through verification).
- Click Next.
- Under Contact Information, enter an Email address where you can be notified about any changes to your project, then click Next.
- Under Finish, review the Google API Services User Data Policy and if you agree, select I agree to the Google API Services: User Data Policy, then click Continue.
- Click Create.
- Link Your Copied Script to Your GCP Project:
- In the Google Cloud console, go to Menu > IAM & Admin > Settings. Go to IAM & Admin Settings
- In the Project number field, copy the value.
- Open your copy of the Google Sheet.
- Go to Extensions > Apps Script.
- In the Apps Script editor, go to Project Settings (the cog icon ⚙️).
- Under “Google Cloud Platform (GCP) Project”, paste the Project Number and click Set Project.
Your copy of the sheet is now correctly configured and authorised to make requests to the Chat API on your behalf.
Step 3: Run Your First Archive
- Refresh your copied Google Sheet. A new menu named Chat Archiver will appear.
- In the Config tab, paste a Google Chat space ID or full room URL and add your email address (you can share your copy of the Google Sheet with other users who will be able to jump to this step).
- Click Chat Archiver > Archive All Spaces.
- The first time, a dialogue will appear asking for authorisation. Grant the permissions to allow the script to run.
- The script will now run, creating both a JSON file and a Google Doc, and will place their links in the corresponding columns in the sheet.
- (Optional) Click Chat Archiver > Setup 15-Minute Trigger so setup a trigger to update the archive documents every 15 minutes.
Understanding the Outputs
The script produces two distinct files, each with a specific purpose:
- The JSON File: The primary output is a structured JSON file. It takes the chat messages and uses a nested format, which groups replies under their parent message, makes it easier for data analysis with LLMs.
- The Google Doc: Alongside the JSON, the script generates a clean Google Doc. This format is perfect as knowledge for a Gem or as a data source in NotebookLM. Crucially, this document is completely regenerated from the JSON data on every run. This means it is a “read-only” artefact; any manual changes will be overwritten.
Important Considerations and Limitations
While this solution is powerful and robust for many use cases, it’s important to understand its design limitations and potential areas for future improvement.
- Access is User-Based (By Design) – The script runs with the permissions of the user who authorises it. This means it can only access and archive Google Chat spaces that the user is currently a member of.
- Future Improvement: For a true, organisation-wide backup solution, this script could be adapted to use a Service Account. With domain-wide delegation granted by a Workspace administrator, a service account could access any chat space in the domain, regardless of membership. This is a more complex setup but is the standard for enterprise-level automation.
- Archives are Text-Only – This solution is focused on archiving the textual content of conversations. It does not save file attachments like images, PDFs, or videos. The JSON data will note that a message contained an attachment, but it won’t contain the file itself.
- Data is Append-Only – The script is designed to add new messages to the archive. It does not synchronise edits or deletions. If a message is edited in Google Chat after it has been archived, the JSON file will still contain the original text. If a message is deleted, it will remain in the archive.
- Apps Script Execution Limits – Google Apps Script has built-in limits to prevent abuse.
- Execution Time: A single script run is limited to 6 minutes for standard Gmail accounts or 30 minutes for Google Workspace accounts. For an exceptionally large space (many hundreds of thousands of messages), the very first archive could potentially time out. Using the “Archive Start Date” field is the best way to manage very large initial backfills.
- File Size: There is a 50 MB limit on the size of a data blob that Apps Script can create. As the JSON archive grows, a single file may eventually hit this limit. For extremely large spaces, the script could be modified to create new files on a yearly or quarterly basis.
- Not a Compliance Substitute for Google Vault – For organisations that require eDiscovery, legal holds, and retention policies for compliance reasons, Google Vault is the official, authoritative tool. This script should be seen as a powerful data extraction and analysis tool, not a substitute for an official compliance solution.
Ideas for Your Archive
Now that you have your complete chat history in a structured format, what can you do with it? Here are a few ideas to get you started:
- Create a Project FAQ with Gemini: Provide the generated Google Doc or JSON file to Gemini and ask it to synthesise a “Frequently Asked Questions” document about a specific project. It can pull out key decisions, find links to important resources, and identify common questions your team has answered.
- Generate an Infographic Summary: Use a prompt like, “Based on this JSON data, identify the top 5 most active members, the busiest day of the week, and the main topics discussed. Present this as data for an infographic.” You can use the Gemini App ‘canvas’ to create a webpage or feed the structured output into a design tool.
- Onboard New Team Members: Give a new team member the Google Doc archive and ask Gemini to act as a project expert. They can ask questions like, “What was the final decision on the ‘Phoenix’ feature?” or “Summarise the key discussions from last March,” and get instant, context-aware answers.
- Perform Topic and Sentiment Analysis: The structured JSON file is perfect for programmatic analysis. You could ask Gemini to write a simple script (or use a Colab notebook) to parse the messages, perform sentiment analysis over time to track team morale, or use natural language processing to identify recurring topics and pain points.
- Build a Project Timeline: Ask Gemini to scan the archive for key dates, deadlines, and project milestones mentioned in the chat, then format the results as a timeline or a table that you can use for project retrospectives and reports.
Summary
Ultimately, this tool is about providing an easy way for you to unlock the value hidden in your team’s conversations. By moving beyond the current limitations and including every message, you create a better true source of truth for analysis and reporting. While we hope future updates to Gemini for Workspace will make this kind of deep integration seamless, for now, this solution gives you more control over your data and a process you can experiment with. I hope this guide helps you get started quickly and if you would like to explore enterprise level solutions get in touch.
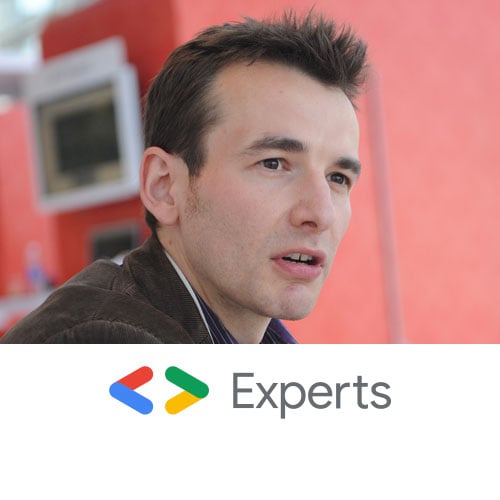
Member of Google Developers Experts Program for Google Workspace (Google Apps Script) and interested in supporting Google Workspace Devs.